Context switching
Advanced feature
In a single account, any number of chatbot projects can be created.
This article will explain:
- What a bot stack is.
- How to switch and return the context.
- Other usage notes on this feature.
Bot stack
For each bot deployed in Tovie Platform, a number of resources is allocated for switching the context to other bots. Bots participating in context switching are organized in a stack.
If the bot script does not involve context switching, its stack always remains the same. Changing the bot context can be achieved in two ways:
- Context switch: the dialog context is passed to another bot. This other bot is pushed onto the bot stack.
- Context return: the last bot is popped from the stack, and the context is returned to the previous bot.
When the context is changed, the bot changes its mode of operation as if it were another bot. However, the communication channel remains the same. This allows implementing complex behavior for interacting with clients.
Context switch
To switch the context from the bot script, push an object with the following properties to $response.replies
.
Property | Type | Required | Description |
---|---|---|---|
type | String | Yes | The type of the reply in the $response.replies array. Must be equal to "context-switch" . |
targetBotId | String | Yes | The ID of the bot where context should be switched. You can find it in the properties of the channel where the bot is deployed. |
targetState | String | No | The absolute path to the state where a transition will be made after switching the context. If you don’t pass preMatch will be executed by default when switching. |
parameters | Object | Yes | Arbitrary data that needs to be passed to another bot. After switching the context, this object will be available in $request.data . |
state: SwitchToFrench
q!: * (switch to/speak) French *
script:
$response.replies = $response.replies || []; // `$response.replies` array setup
$response.replies.push({
type: "context-switch",
targetBotId: "13721-frBot-13721-BkN-41730",
targetState: "/Start",
parameters: {}
});
Context return
After the context has been switched, the script of the previous bot is no longer available. The context must be explicitly returned from the new bot script to the script where switching took place.
To return the context to the previous bot script, push an object with the following properties to the $response.replies
array.
Property | Type | Required | Description |
---|---|---|---|
type | String | Yes | The type of the reply in the $response.replies array. Must be equal to "context-return" . |
state | String | No | The absolute path to the state where a transition will be made after switching the context. If you don’t pass state , the request will be processed in the root theme / after the context has been returned. The appropriate state will be selected at runtime. |
data | Object | Yes | Arbitrary data that needs to be passed to another bot. After returning the context, this object will be available in $request.data . |
state: SwitchToEnglish
q!: * (switch to/speak) English *
script:
$response.replies = $response.replies || []; // `$response.replies` array setup
$response.replies.push({
type: "context-return",
state: "/Start",
data: {}
});
Usage notes
Stack overflow
Theoretically, switching the context back to the previous bot can be done with context-switch
. However, this usage is incorrect, because the same bot will be pushed onto the stack multiple times.
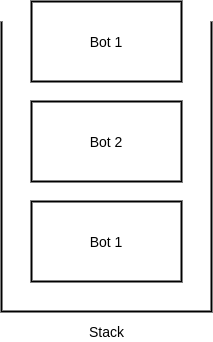
context-return
must be used for returning the context.Event processing
If the context is switched from an example bot 1 to an example bot 2, all requests from the client will be processed in the context of bot 2.
However, if a timeout or an event which can be processed in bot 1 occurs in the channel, the event will be processed in the appropriate state. The context will be automatically returned to bot 1.
$pushgate
built-in service methods
and does not apply to system events.noContext
flag for the state where the event is handled.Learn how to use context switching in practice by walking through the multilingual bot tutorial.
Handler execution in context switch
If you transitioned to the second bot’s state using context switch, all relevant handlers from the second bot’s script will be executed by default, except preMatch
.
You can also change this behavior for each handler using the allowedInContextSwitch
argument of the bind
function.
The allowedInContextSwitch
argument is applied only when the handlers are called for the first time after the transition to the second bot.